Never miss an error in Ionic
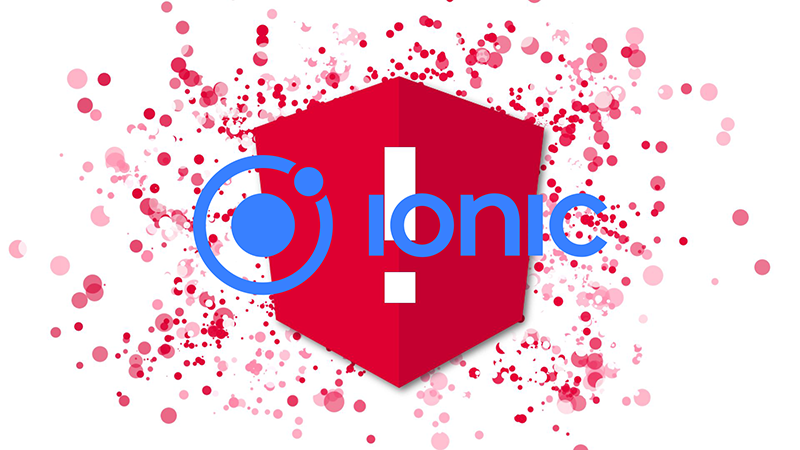
You may know that Angular provides a hook
for centralized exception handling that you may override. The default implementation prints error messages to the console
.
It’s usual for developers to override the default implementation by providing their own ErrorHandler
. By doing this, they can manage all the exceptions and do something with them (i.e. store them in some database…).
The way to do this is pretty straightforward:
class CustomErrorHandler implements ErrorHandler {
handleError(error: Error) {
// do something with the exception
}
}
@NgModule({
providers: [{ provide: ErrorHandler, useClass: CustomErrorHandler }],
})
class AppModule {}
Whenever an unhandled exception happens you would expect it to be logged into the console
, regardless your custom implementation doesn’t do it explicitly. At least this is what the default Angular implementation provides and, indeed, that’s the way it works. Check it out live in this StackBlitz project.
But… what about Ionic?
In Ionic, in order to provide the same behavior you would tipically extend the IonicErrorHandler:
class CustomErrorHandler extends IonicErrorHandler {
handleError(error: Error) {
// do something with the exception
}
}
@NgModule({
providers: [{ provide: ErrorHandler, useClass: CustomErrorHandler }],
})
class AppModule {}
So far so good but, unfortunately, if you forget to explicitely call console.error
, you won’t see anything in your console
. In this case, the default Angular implementation won’t work and you will totally miss the error.
You can take a look at this StackBlitz project and check the console
out. You won’t see any errors and the screen will remain empty, because, in this case, the error happens in the constructor of one of the app.component
’s depencencies.
tl;dr
If you’re working with Ionic and you override the default IonicErrorHandler, don’t forget to explicitly call console.error
in that handler if you want to have some visibility in the console
in case some unexpected error happens .